Understanding Docker Networking: A Comprehensive Guide
Docker is an open-source platform that enables developers to automate the deployment of applications inside lightweight, portable containers. These containers include everything needed to run an application, ensuring consistency across development, testing, and production environments.
Docker networking is a fundamental aspect of this ecosystem, enabling seamless communication between containers, services, and external networks. It allows developers to connect and isolate containers while ensuring optimal performance, scalability, and security. This guide explores the concepts, types, and practical implementation of Docker networking.
What is Docker Networking?
Docker networking is the system that facilitates communication between Docker containers and other entities, such as external systems or the host machine. Containers operate in isolated environments, and networking enables them to exchange data efficiently. Docker’s networking capabilities provide flexibility for various deployment scenarios, from small-scale projects to large-scale distributed systems.
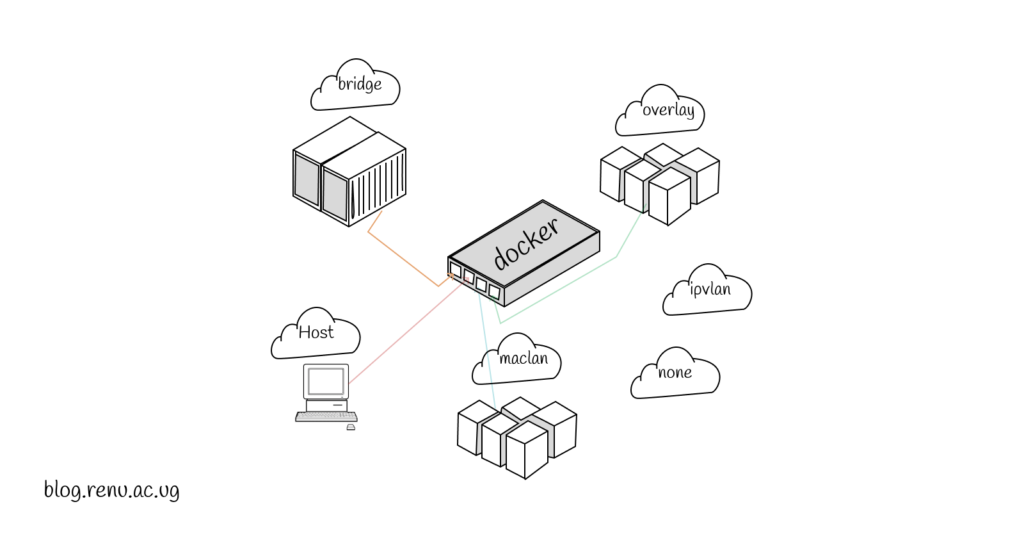
Types of Docker Networks
Docker offers several networking options to cater to diverse use cases:
Drivers: The following network drivers are available by default, and provide core networking functionality:
Driver | Description |
---|---|
bridge | The default network driver. |
host | Remove network isolation between the container and the Docker host. |
none | Completely isolate a container from the host and other containers. |
overlay | Overlay networks connect multiple Docker daemons together. |
ipvlan | IPvlan networks provide full control over both IPv4 and IPv6 addressing. |
macvlan | Assign a MAC address to a container. |
1. Bridge Network
The default network mode for Docker containers, the bridge network, is used when no specific network is defined. It allows containers on the same host to communicate with each other while remaining isolated from external systems unless explicitly configured.
Illustration: Imagine a local network within a single building where computers communicate but are isolated from the internet without specific configuration.
Key Features:
- Containers can communicate using container names.
- Suitable for standalone applications.
- Requires port mapping to expose services to external systems.
Example:
# Run a container in the default bridge network
$ docker run -d --name my-container nginx
2. Host Network
In the host network mode, the container shares the host’s networking stack, eliminating the network isolation layer. This is useful for performance-sensitive applications that require direct access to the host’s network.
Illustration: Think of a container merging directly into the host’s operating system, as if it were another process running natively on the host.
Key Features:
- No isolation between the container and host.
- Bypasses NAT (Network Address Translation).
- Suitable for high-performance applications like gaming servers.
Example:
# Run a container using the host network
$ docker run --network host nginx
3. Overlay Network
Overlay networks are used in multi-host scenarios where containers across different hosts need to communicate securely. These networks rely on a Docker Swarm or similar orchestration tools to manage connectivity.
Illustration: Visualize a virtual private network (VPN) that connects computers across different geographic locations securely.
Key Features:
- Facilitates communication across multiple Docker hosts.
- Secure and scalable.
- Ideal for distributed applications and microservices.
Example:
# Create an overlay network
$ docker network create -d overlay my-overlay-network
4. Macvlan Network
Macvlan networks assign a MAC address to each container, making it appear as a physical device on the network. This network type is ideal for scenarios where containers need to be directly accessible from the external network.
Illustration: Imagine each container as an individual computer directly plugged into a network switch.
Key Features:
- Containers act as independent devices on the network.
- Suitable for legacy applications requiring direct network integration.
Example:
# Create a Macvlan network
$ docker network create -d macvlan \
--subnet=192.168.1.0/24 \
--gateway=192.168.1.1 \
-o parent=eth0 my-macvlan-network
5. None Network
The none network disables all networking for a container, ensuring complete isolation. This is useful for specific use cases like batch jobs or containers that do not require networking.
Illustration: Picture a computer disconnected from any network, functioning independently without external communication.
Example:
# Run a container with no network
$ docker run --network none busybox
Key Concepts in Docker Networking
Container-to-Container Communication
- Containers on the same network can communicate using their names or IP addresses.
- Use the
--link
option (deprecated) or a custom network for better naming and resolution.
External Communication
- Containers can access external systems via the host’s network interface.
- Use port mapping (
-p
or--publish
) to expose container services.
DNS Services
Docker networks include an embedded DNS server, allowing containers to resolve names of other containers on the same network.
Network Drivers
Network drivers, such as bridge
, overlay
, and macvlan
, define the underlying implementation of Docker networks.
Managing Docker Networks
Listing Networks
To view all available Docker networks:
$ docker network ls
Inspecting Networks
To get detailed information about a specific network:
$ docker network inspect <network-name>
Creating a Custom Network
To create a user-defined network:
$ docker network create my-custom-network
Connecting a Container to a Network
To attach an existing container to a network:
$ docker network connect my-custom-network my-container
Disconnecting a Container from a Network
To detach a container from a network:
$ docker network disconnect my-custom-network my-container
Best Practices for Docker Networking
- Use User-Defined Networks: Default bridge networks lack advanced features like automatic DNS resolution. Use custom networks for better control and isolation.
- Restrict External Access: Avoid exposing unnecessary ports to minimize security risks.
- Implement Network Policies: Use tools like Calico or Weave to enforce network policies and ensure secure communication.
- Monitor Network Traffic: Use monitoring tools to track traffic and detect anomalies.
- Leverage Service Discovery: Use Docker’s built-in DNS for service discovery in multi-container applications.
Networking and Docker Compose
Docker Compose simplifies the process of defining and managing multi-container Docker applications. Networking plays a critical role in Compose by connecting services defined in the docker-compose.yml file. Each service can communicate with others seamlessly through an automatic network created by Compose.
Key Features:
- Automatic Network Creation: When docker-compose up is executed, Compose creates a default bridge network for the services.
- Service Discovery: Each service can be accessed by its name as defined in the Compose file. Compose leverages Docker’s built-in DNS server for resolution.
- Custom Networks: Compose allows defining custom networks for better control and segmentation of services.
Example docker-compose.yml:
version: '3.9'
services:
app:
image: my-app
networks:
- custom-network
db:
image: mysql
environment:
MYSQL_ROOT_PASSWORD: example
networks:
- custom-network
networks:
custom-network:
driver: bridge
Conclusion
Docker networking is a versatile and powerful feature that bridges the gap between containerized applications and the external world. By understanding the various network types and their use cases, you can design scalable, secure, and efficient containerized environments. Whether you’re building a small application or a distributed microservices architecture, mastering Docker networking is essential for modern DevOps and development workflows.